This is an unofficial api wrapper for TikTok.com in python. With this api you are able to call most trending and fetch specific user information as well as much more.
This api is designed to retrieve data TikTok. It can not be used post or upload content to TikTok on the behalf of a user. It has no support for any user-authenticated routes, if you can't access it while being logged out on their website you can't access it here.
These sponsors have paid to be placed here or are my own affiliate links which I may earn a commission from, and beyond that I do not have any affiliation with them. The TikTokAPI package will always be free and open-source. If you wish to be a sponsor of this project check out my GitHub sponsors page.
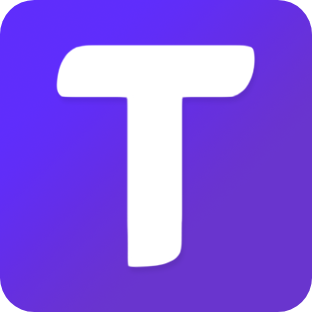
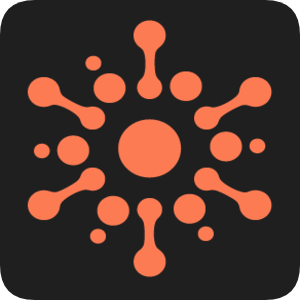
Trusted by the major influencer marketing and social media listening platforms.
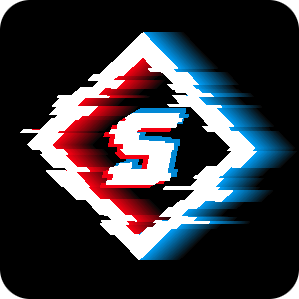
Scale your TikTok automation and get unblocked with SadCaptcha.
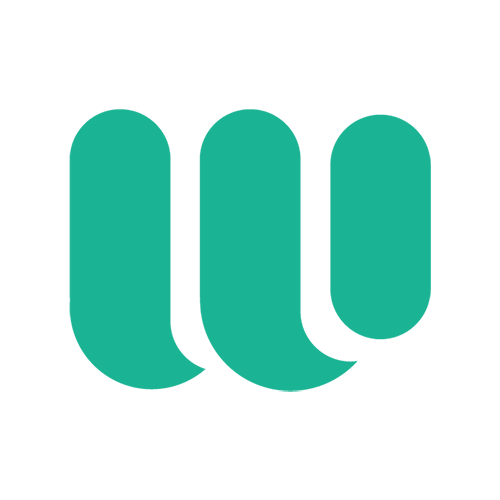
You can find the full documentation here
To get started using this API follow the instructions below.
Note: If you want to learn how to web scrape websites check my free and open-source course for learning everything web scraping
- Star the repo 😎
- Consider sponsoring me on GitHub
- Send me an email or a LinkedIn message telling me what you're using the API for, I really like hearing what people are using it for.
- Submit PRs for issues :)
Note: Installation requires python3.9+
If you run into an issue please check the closed issues on the github, although feel free to re-open a new issue if you find an issue that's been closed for a few months. The codebase can and does run into similar issues as it has before, because TikTok changes things up.
pip install TikTokApi
python -m playwright install
If you would prefer a video walk through of setting up this package YouTube video just for that. (is a version out of date, installation is the same though)
If you want a quick video to listen for TikTok Live events in python.
Clone this repository onto a local machine (or just the Dockerfile since it installs TikTokApi from pip) then run the following commands.
docker pull mcr.microsoft.com/playwright:focal
docker build . -t tiktokapi:latest
docker run -v TikTokApi --rm tiktokapi:latest python3 your_script.py
Note this assumes your script is named your_script.py and lives in the root of this directory.
-
EmptyResponseException - this means TikTok is blocking the request and detects you're a bot. This can be a problem with your setup or the library itself
- you may need a proxy to successfuly scrape TikTok, I've made a web scraping lesson explaining the differences of "tiers" of proxies, I've personally had success with webshare's residential proxies (affiliate link), but you might have success on their free data center IPs or a cheaper competitor.
-
Browser Has no Attribute - make sure you ran
python3 -m playwright install
, if your error persists try the playwright-python quickstart guide and diagnose issues from there. -
API methods returning Coroutine - many of the API's methods are async so make sure your program awaits them for proper functionality
Here's a quick bit of code to get the most recent trending videos on TikTok. There's more examples in the examples directory.
Note: If you want to learn how to web scrape websites check my free and open-source course for web scraping
from TikTokApi import TikTokApi
import asyncio
import os
ms_token = os.environ.get("ms_token", None) # get your own ms_token from your cookies on tiktok.com
async def trending_videos():
async with TikTokApi() as api:
await api.create_sessions(ms_tokens=[ms_token], num_sessions=1, sleep_after=3, browser=os.getenv("TIKTOK_BROWSER", "chromium"))
async for video in api.trending.videos(count=30):
print(video)
print(video.as_dict)
if __name__ == "__main__":
asyncio.run(trending_videos())
To directly run the example scripts from the repository root, use the -m
option on python.
python -m examples.trending_example
You can access the full data dictionary the object was created from with .as_dict
. On a video this may look like
this. TikTok changes their structure from time to time so it's worth investigating the structure of the dictionary when you use this package.
All changes will be noted on V6.0.0 if you want more information.
- Maintainability
- Was getting difficult to maintain with how TikTok was directly detecting HTTP requests.
- Switched to using a pool of async playwright pages to make it more difficult to detect, and hopefully easier to maintain in the future
- Async
- It's been asked for a lot, and now fully async needed especially since the package is using a pool of playwright instances
- User Flexibility
- Added a lot of function argument options that should be much more explicit than what was done previously.
- Hopefully if something is broken there's a workaround without having to modify the package itself (although if you do notice an issue please open an issue or submit a PR)
- Documentation
- Goes with user flexibility a little bit but switched over to sphinx documentation for more explicit content
- If you run into an issue or are confused please open a github issue or submit a PR to fix documentation, it's always welcome! 🤠
The biggest change is that everything is now async. You can see above how you might want to call an async function in python as well as the examples directory for more examples.